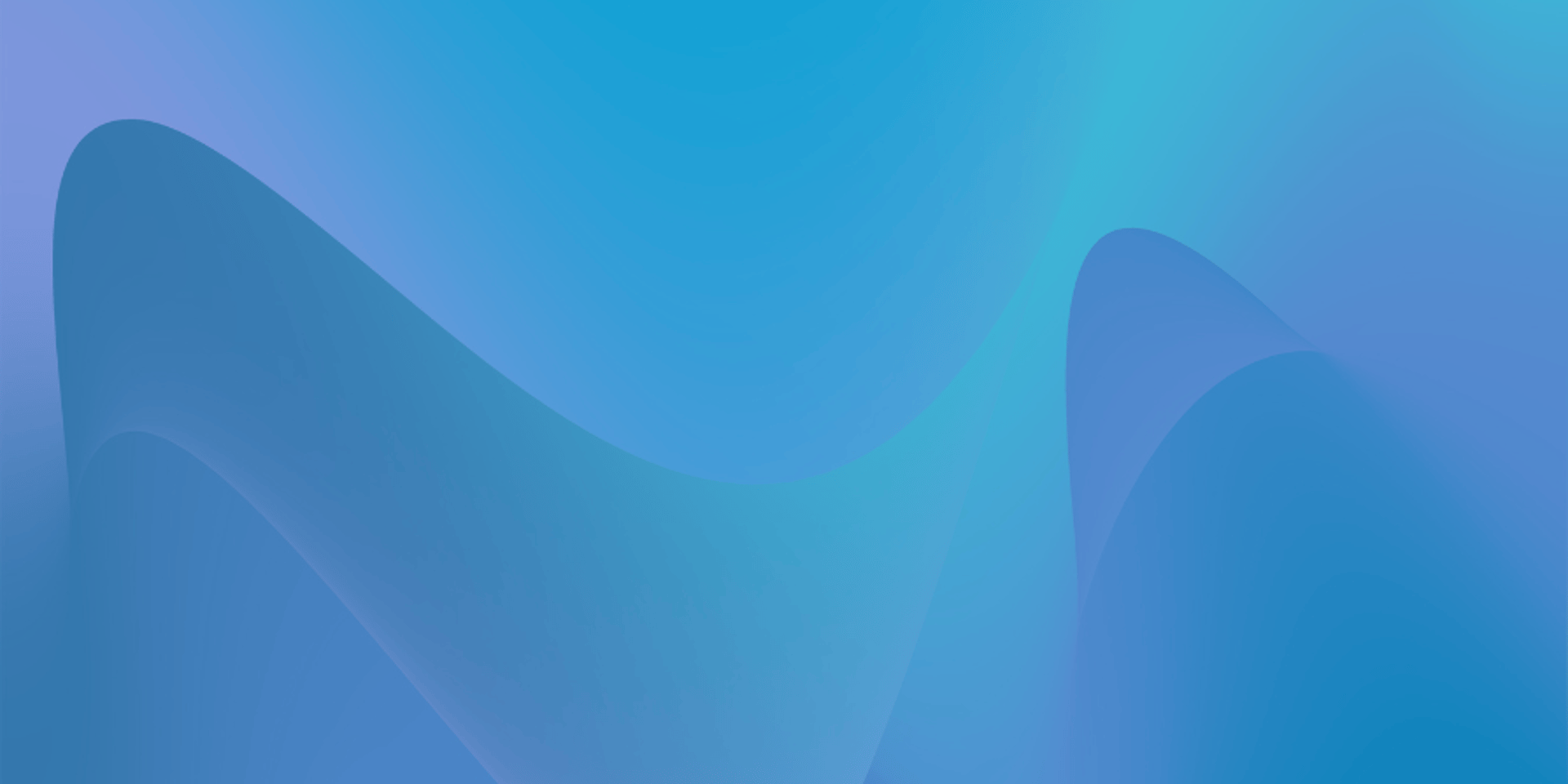
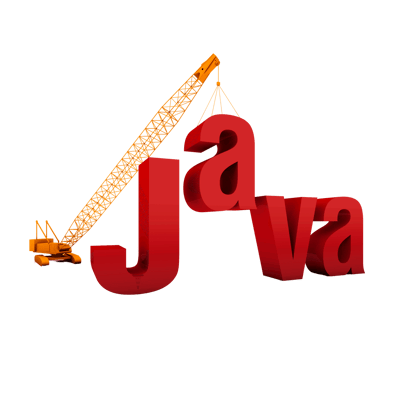
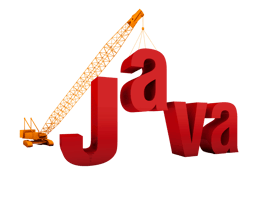
Certified Java Developer
Duration: 108 Hours | 3 Months
A Certified Java Developer specializes in building robust, scalable, and efficient Java applications. This role involves designing and developing software solutions, debugging code, optimizing performance, and ensuring seamless system integration. Java developers work with frameworks like Spring and Hibernate, manage databases, and implement secure coding practices. The program focuses on core Java concepts, object-oriented programming (OOP), multithreading, data structures, APIs, and enterprise applications to equip learners with industry-relevant skills.
Prerequisites
Basic understanding of programming concepts is recommended.
Familiarity with object-oriented principles is advantageous but not mandatory.
Strong analytical thinking and problem-solving skills are essential for success in this program.
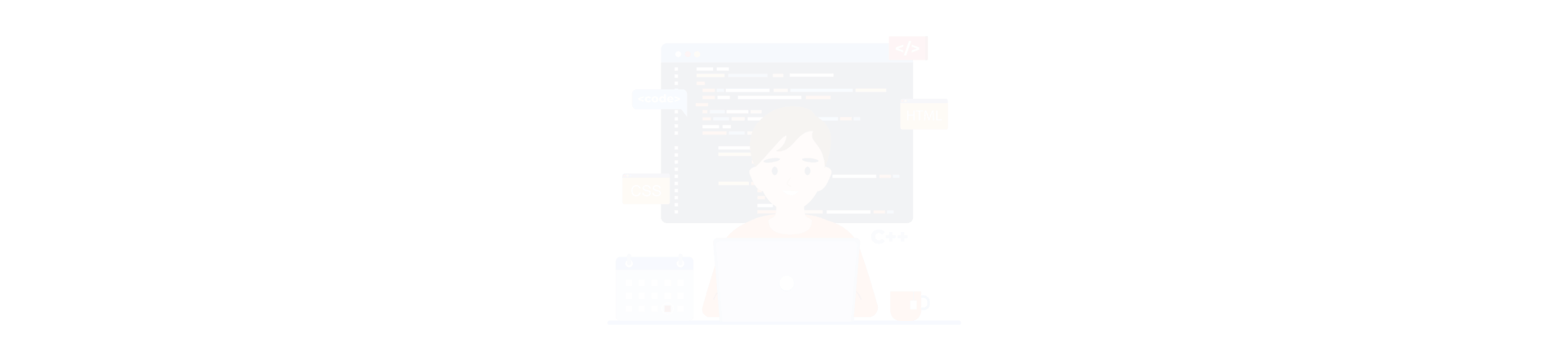


Course Objectives
The Certified Java Developer Training Program is designed to equip participants with the skills to develop, deploy, and maintain Java-based applications. The primary objective is to enable learners to build robust, scalable, and secure software solutions while mastering core Java concepts, object-oriented programming, and advanced development techniques.
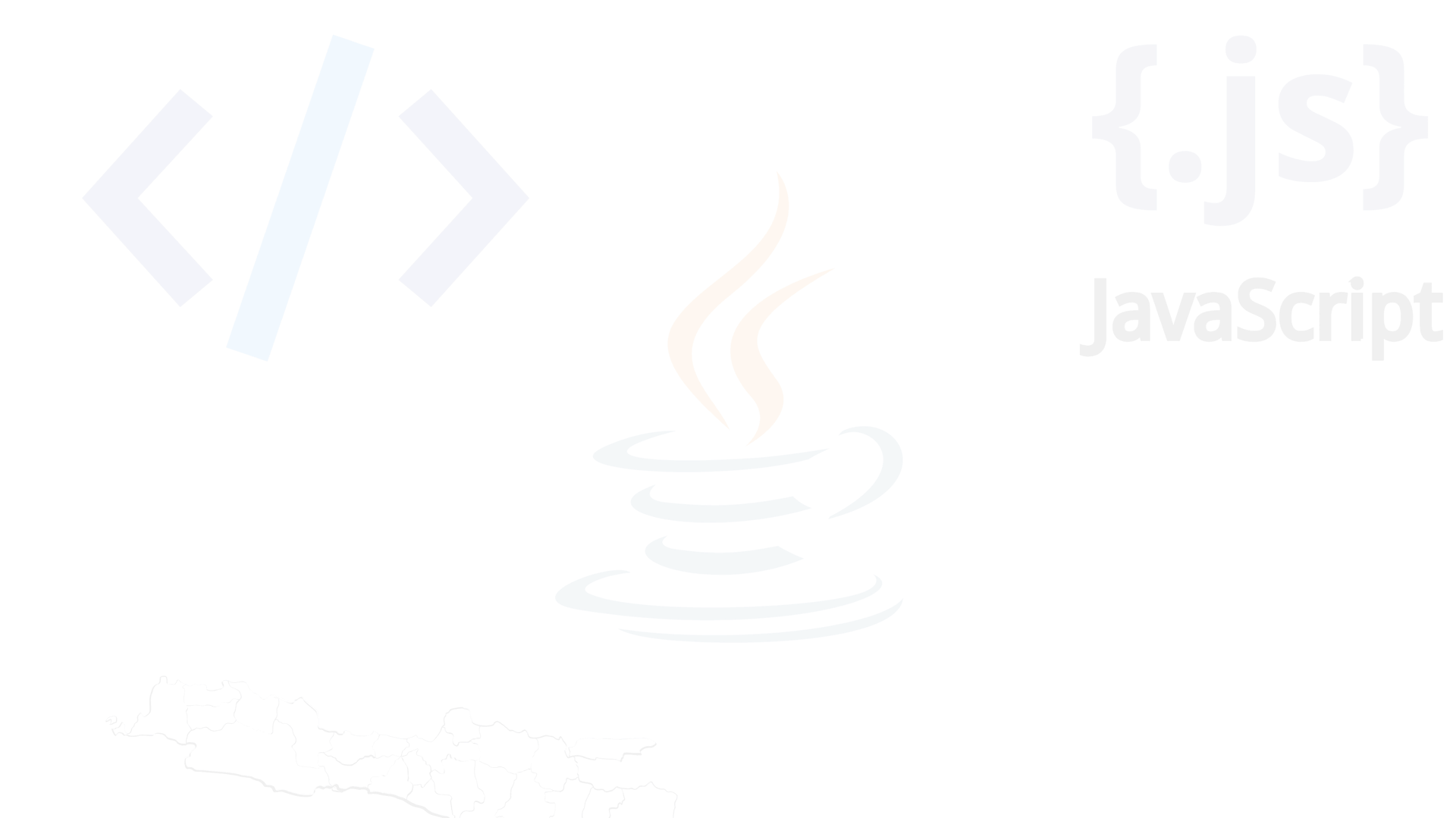
What You Will Learn
_______________________________________________
Our curriculum offers practical knowledge and hands-on experience to master key aspects of Java development. Here's what you will learn throughout the course:
Core Java
Java Syntax & Data Types
Variables & Operators
Control Flow Statements
Loops (For, While, Do-While)
Methods & Functions
Object-Oriented Programming (OOP)
Classes & Objects
Inheritance & Polymorphism
Abstraction & Encapsulation
Interfaces & Abstract Classes
Data Structures & Algorithms
Arrays & Strings
Linked Lists, Stacks, and Queues
Trees & Graphs
Sorting & Searching Algorithms
Recursion & Dynamic Programming
Exception Handling
Try, Catch, Finally
Checked & Unchecked Exceptions
Custom Exceptions
File Handling & I/O
Reading & Writing Files
Serialization & Deserialization
BufferedReader & Scanner
Multi-threading & Concurrency
Thread Lifecycle
Synchronization & Locks
Executor Framework
Parallel Processing
Collections Framework
List, Set, Map
HashMap vs TreeMap vs LinkedHashMap
Iterators & Streams
JDBC (Java Database Connectivity)
Connecting to Databases
CRUD Operations
Connection Pooling
Spring & Hibernate
Spring Boot Essentials
Dependency Injection
Hibernate ORM & JPA
REST API Development
Testing & Debugging
JUnit & TestNG
Debugging Tools in IDEs
Logging with Log4j
Build Tools & Version Control
Maven & Gradle
Git & GitHub Integration
Microservices & Cloud
Spring Boot Microservices
Docker & Kubernetes
AWS & Azure Basics
Security in Java
Encryption & Hashing
Secure Coding Practices
Authentication & Authorization
Professional Development
Java Certifications (OCJP, OCA)
Contributing to Open Source
Building Java Projects
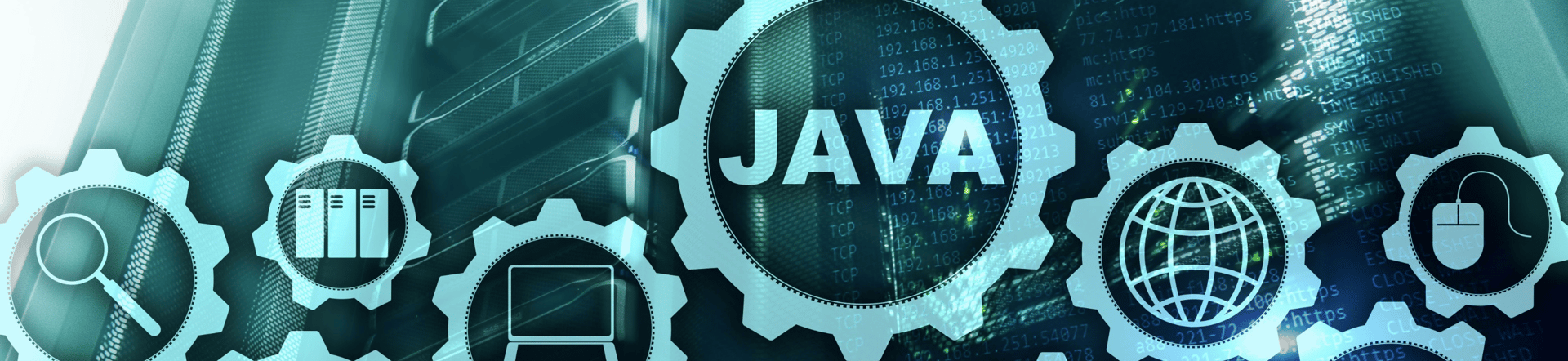